Using Background Programs
A Background Program (BP) is a collection of steps that will execute on the LI-6800 console “in the background” to accomplish various tasks. These steps can be put together with tools provided on the console’s user interface.
While BPs can do the same things as conventional programs, they have three significant advantages: 1) you can make your own BPs, 2) the scope of what can be done via a BP exceeds what can be done with a program, 3) any number of BPs can be run simultaneously.
Some background information will help you be successful with background programs.
Nomenclature and symbols
We use some terminology and some highlights in this document that are explained here.
- Program - The traditional LI-6800 method of running automated logging, etc. Called Auto Programs in software older than 2.0.
- BP - Background Program. The subject of this document
- Open, New, Start, etc. - Refers to a button on the interface.
- Open/New, Build, Set, Monitor - Refers to a tab label, or the screen associated with that tab label.
- LOOP, SETCONTROL, WAIT - A BP step.
- LOOP [Duration] - A BP step of a particular sub-type.
Python
Even through BPs are Python files, you do not need to be a Python programmer to build or run them, since the LI-6800 software provides a graphical user interface for doing those tasks. However, knowing a little bit of Python syntax can be very helpful if you wish to design your own BPs and take advantage of some powerful options that are available. The BP interface contains places where you are invited to type in values, expressions, variable names, etc., and even if you have no inclination to become a programmer, some simple knowledge can be very useful to you. For example, rather than entering in a list of setpoints for light intensity, you might instead type in something like randomList(50,2000,15)
, which, when the program runs, will generate a randomized list of 15 linearly spaced setpoints ranging between 50 and 2000.
If you are not a programmer, the next section is for you.
If you don't know Python
The Python world consists of objects of various types, such as numbers, strings, lists, and so on. You create and keep track of your objects by assigning them to variables. Consider these simple examples of Python assignments:
a = 10
a points to an integer.ByeBye = 'this is a string'
ByeBye points to a string.c66 = ["hello", 55, ByeBye]
c66 points to a list containing a string, number, and string.dd = True
dd points to a Boolean.e_xy = ByeBye+" and " + c66[0]
e_xy will be the string 'this is a string and hello'.fff = 'file '+str(a)
fff makes a string out of a number, so is = 'file 10'.hh = a >15 and dd
hh will be False, since a is not greater than 15.
Variable names should start with a letter (a-z or A-Z), and can contain numbers and underscores (_). Python is case sensitive. There are some keywords you should avoid when creating variable names (Figure 12‑1), but if you accidentally use one, you will be notified when you run the program and you can fix it.
The same name rule also applies to functions. A function is a named collection of instructions that can be invoked by using the name followed by a list of zero or more arguments (information passed to the function). Examples:
doSomething(a,5,c66)
Calls the function doSomething, sending to it three arguments.tryThis()
Runs the function tryThis which takes no arguments.fff = 'file '+str(a)
The function str() makes a string out of a number, so fff becomes 'file 10
' since a is 10.
Some functions only exist for a particular type of object. For those, we use a dot: object.function(). For example, strings have a replace(x, y) function that replaces all occurrences of x with y in that string. So, if the string pointed at by fff is 'file 10', then
gg = fff.replace('file','cabinet')
gg becomes 'cabinet 10'
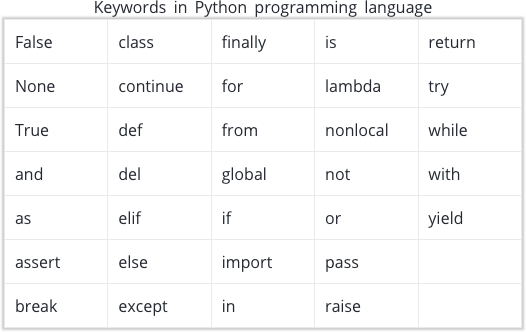
Pay attention to the hints
The interface for configuring steps in a BP frequently contain hints that tell you what is expected to configure that step. For example, the configuration interface for the BP step ASSIGN, which lets you create a variable and assign it to an expression (or other things), is shown in Figure 12‑2.
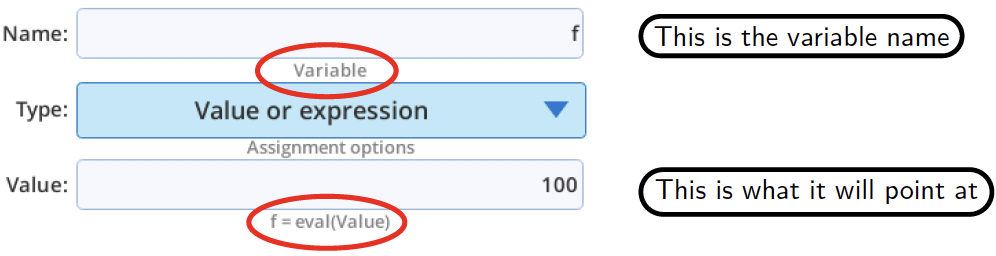
When an edit box has a hint with 'eval()' in it, it means that entry is going to be passed to Python's eval() function when the program runs. This allows your entry to be more complicated than, say, a numeric value. In the example above, the variable is going to be assigned to whatever the eval() returns, regardless of type. In other settings (Figure 12‑3), a specific type is required, and that is reflected in the hint. In the first example below, the entry is 10. It could just as easily be an expression, like 8+2
, or (if you have defined variables x and y) (x+y)/3.14159
, or anything that evaluates to something numeric.
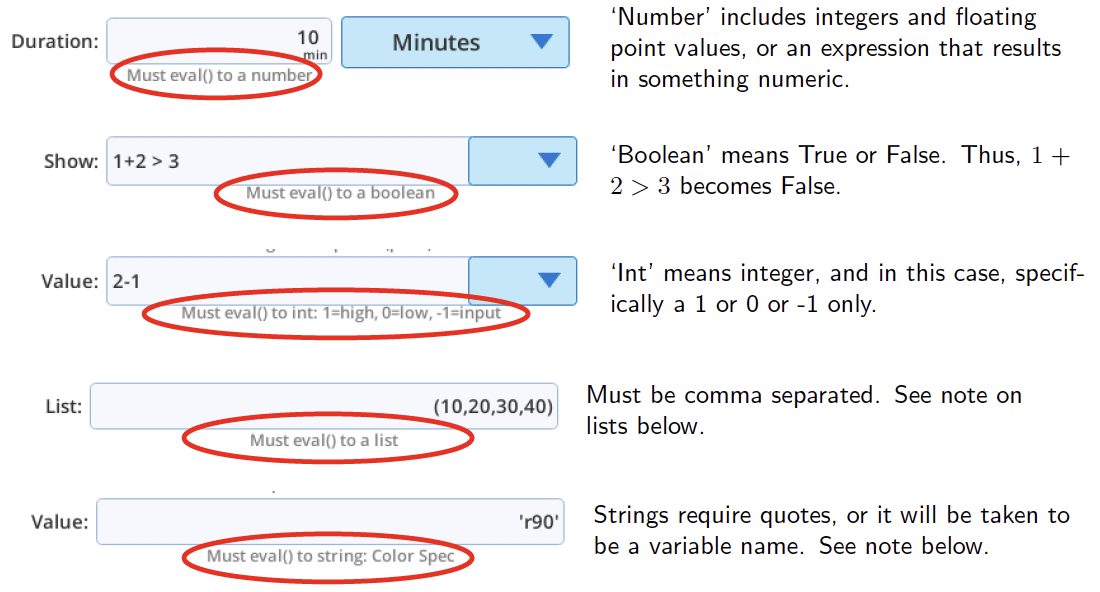
eval()
in the hint.Lists: "Must eval() to a list" means to enter a sequence that is comma separated. You can use [], or (), or leave them off:
[1,2,3] or (1,2,3) or 1,2,3
In Python, [1,2,3]
is a list that can be modified; otherwise, it is a tuple, a list that cannot be modified. For lists you enter in a BP interface, that distinction is usually not important. To enter a single valued list, use a trailing comma.
[1,] or (1,) or 1,
Trailing commas like this (1,2,3,)
are always allowed, regardless of the size of the list.
Strings: Take special note of the string example in Figure 12‑3. If you are being asked for a string, such as a color, or a file name, that will be passed to eval(), and you enter
/home/licor/myfile.txt
eval() will treat that entry like a variable name and you'll see an error. To avoid that, put single or double quotes around it.
'/home/licor/myfile.txt' or "/home/licor/myfile.txt"
The reason for this is to allow for variable names. For example, you might wish to programmatically name a new file each time through a loop, so you enter
'/home/licor/myfile'+str(count)+'.txt'
where count is some variable you have defined, giving you a new file each time through:
/home/licor/myfile0.txt
/home/licor/myfile1.txt
/home/licor/myfile2.txt
Failure to follow the guidance provided in the hint will usually result in an error (Figure 12‑4).
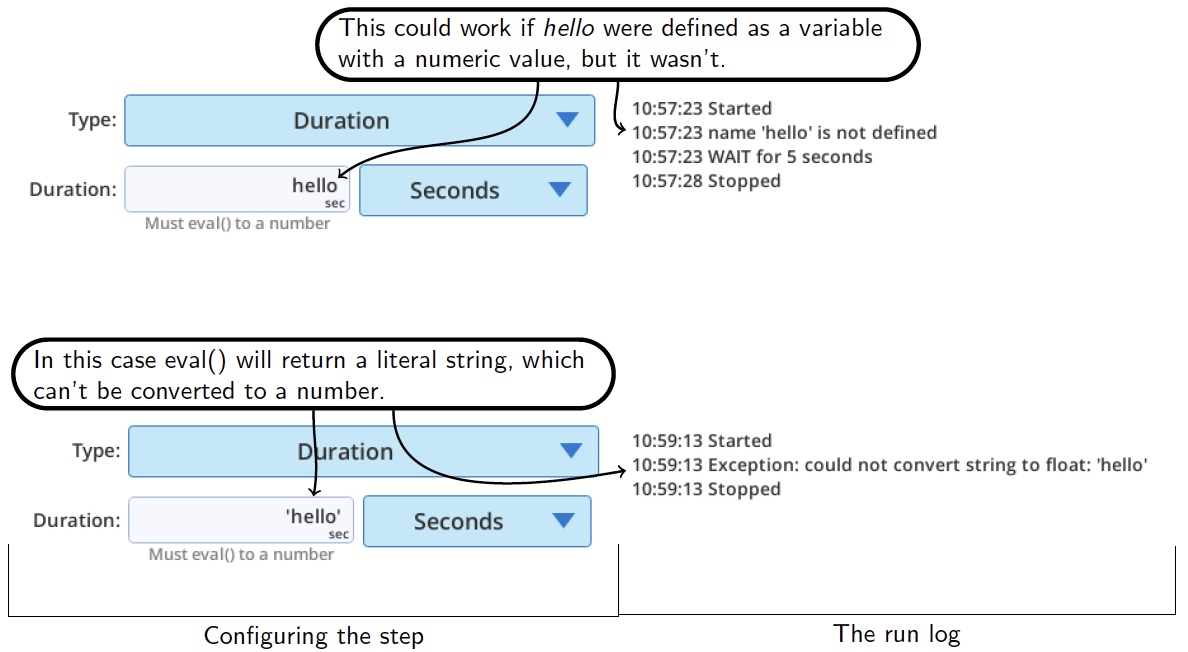
If you do know Python
BP files are Python files (.py). A simple one from one of the upcoming examples is shown in Listing 12‑1.
Listing 12‑1. Listing of the file /home/licor/apps/examples/VariableLogInt.py.
from bpdefs import ASSIGN, LOOP, SETCONTROL, LOG, Nothing, CheckBox, Text, Button, DropDown, RadioBtns, EditBox
steps=[
# Assign a variable to an expression: ASSIGN('varname', exp="expression" [,dlg=Nothing()])
ASSIGN("logint",
exp="lambda x: 30/(1+50*math.exp(-0.03*x))+0"),
# Assign a variable to an expression: ASSIGN('varname', exp="expression" [,dlg=Nothing()])
ASSIGN("test",
exp="lambda x: x if x >= 1 else 0"),
# Loop through a list: LOOP(list=itemList [,var=varname] [,mininc=''])
LOOP(list="1500,50,1500",
var="x",
steps=(
# Set a control:
SETCONTROL('target', 'value', 'eval' [,opt_target=''])
SETCONTROL("Qin","x","float"),
# Loop for a duration: LOOP(dur="float"
[,units='Seconds' Second|Minutes|Hours ] [,var=''] [,mininc=''])
LOOP(dur="15",
units="Minutes",
var="t",
mininc="test(logint(t))",
steps=(
# Log a data record:
LOG([avg='Default'] [,match='Default] [,flr='Default'] [flash='Default'])
LOG(avg="Off",
match="Off",
flr="0: Nothing"),
)
),
)
),
]
A BP file contains one list, which is always named steps
. Each item in steps
is created using a constructor, with class names such as ASSIGN, LOOP, LOG, etc. The line before each constructor contains a comment, showing the options available for the version of constructor used.
The first thing to know is this: running a BP is not simply a matter of running the .py file; rather, the .py file is compiled (eval()) to obtain a list of program steps (steps). The thread that actually executes when a BP uses steps as data as it constructs itself.
A second thing to note is that because we are just constructing data in a list, variables and expressions have to exist at this stage as strings, sometimes even doubly-quoted strings. The first parameter in ASSIGN, for example, is destined to become a Python variable (but not yet), so at this stage, it is a quoted string. Similarly, what will be the object assigned to that variable also starts out as a string. In the two ASSIGNs above, the assigned objects will become lambda expressions. In summary, you have to keep in mind that you are writing code that will be sent to a Python's eval() or exec() statements twice: once to build the step, then later when the step processes its parameters.
Because BP files are .py files, they can be written and/or edited outside of the LI-6800 console's user interface using any text editor. Ideally, of course, you should use an editor designed for Python, to eliminate syntax errors in the editing stage.
Consider using VNC
The touch screen keyboard on the LI-6800 console does not lend itself well to typing long expressions and names that may be needed when developing BPs.
Consider connecting to the LI-6800 with a virtual network computing (VNC) viewer client when doing BP development. Clients are available for a wide range of platforms from https://www.realvnc.com/en/connect/download/viewer/
Using a VNC client on your computer allows you to use your computer's keyboard to enter those long expressions and names. But how?
The solution is shown in Figure 12‑5. When the LI-6800 keyboard (or keypad) appears for an entry, the VNC client keyboard will not immediately be “activated”. To do this, you must click in the keypad entry area somewhere to the left of the entry's last character. (If the entry area is empty, you may have first click on a couple of keypad buttons to put some characters there, then click to the left of the last one.)
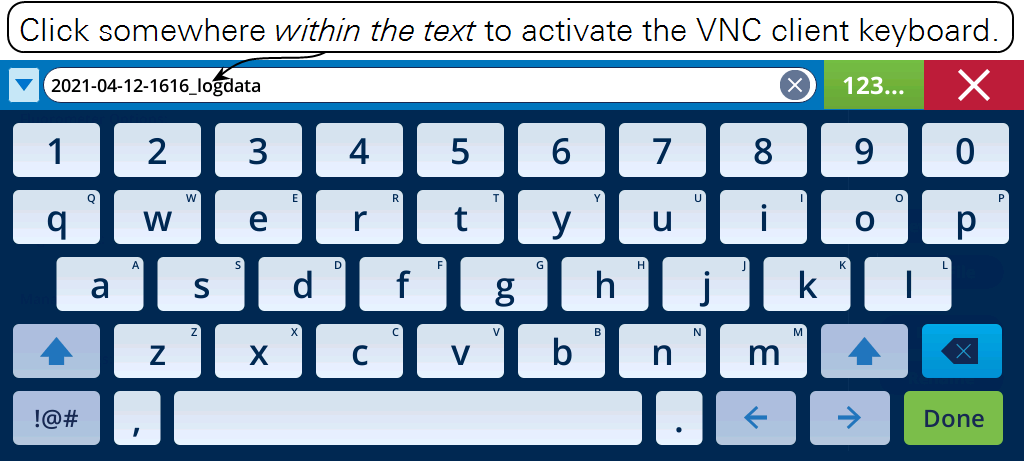